A matplotlib demo#
This notebook demonstrates some plotting features of matplotlib.
# import matplotlib, and some packges to make fake data
import matplotlib.pyplot as plt
import numpy as np
import scipy.stats as stats
Create some fake datasets. First, a couple of PDFs…
# normal pdf x and y values for hills
x_pdf = np.linspace(-3,3,100)
normal_pdf = stats.norm.pdf(x_pdf)*15
normal_pdf2 = stats.norm.pdf(x_pdf*1.5)*20
Now, a couple of sine waves…
# x and y values for blue sine waves
x = np.linspace(0,2*np.pi,1000)
y2 =np.sin(x*3)/2 + 3
y3 =np.sin(x*4)/4 + 3
Plot our data using matplotlib, style and annotate the plot with text.
fig, ax = plt.subplots(nrows=1, ncols=1, figsize=(8,3))
# blue sine waves
ax.fill_between(x,y2, np.zeros_like(y2), color='#377EB8', alpha=0.5, zorder=0)
ax.fill_between(x,y3, np.zeros_like(y3), color='#377EB8', alpha=0.7)
# white sine wave lines
ax.plot(x,y2-0.3, c='#fff', lw=2, zorder=2, alpha=0.7)
ax.plot(x,y3-0.3, c='#fff', lw=2, zorder=2, alpha=0.7)
# green hill pdf
ax.fill_between(x_pdf,normal_pdf, np.zeros_like(normal_pdf), color='#4DAF4A', alpha=0.7, zorder=3)
ax.fill_between(x_pdf,normal_pdf, np.zeros_like(normal_pdf), color='white', alpha=1, zorder=1)
# grey hill pdf
ax.fill_between(x_pdf-2,normal_pdf2, np.zeros_like(normal_pdf), color='grey', alpha=0.7, zorder=2)
# text
ax.text(-1.4,6.5,'Data Analysis', size=28, weight='bold', color='#555555', zorder=4)
ax.text(0.9,5.1,'In Water Sciences', size=17, color='#555555', zorder=4)
# set plot axes limits
ax.set_ylim(-2,9)
ax.set_xlim(-4,12)
# turn off axes lines and labels
ax.axis('off');
# save figure
plt.savefig('logo.svg')
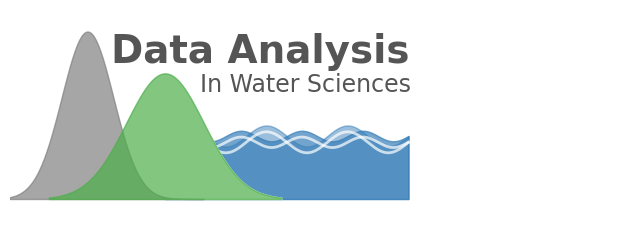